In 2019 React is moving fast to concurrent rendering era. This change will be a massive boost for client-side apps and will help frontend engineers to tackle slow performance bottlenecks. If you want to hear more about concurrent rendering in React check this awesome pitch about it here:
That said, concurrent rendering is the holy-grail and the ultimate vision of the React team but in order to reach there many things need to change first. During the last 6 months we have been witnessing some really big api changes in React 16 and lately we welcomed React hooks in the frontend world.
Other changes before this were:
- React Fragments
- new refs and context apis
- findDOMNode got deprecated
- changes for lifecycle methods
- introduction of getDerivedStateFromProps, getSnapshotBeforeUpdate and componentDidCatch
- announcement that some hooks like componentWillReceiveProps and componentWillMount are considered error-prone so they will be highlighted as unsafe in the upcoming versions of the library
- of course React hooks and many many more…
I know these sound like brand new toys in the hands of a little child for most of us and this is the hungry developer speaking inside me. So what are we doing if we need to make some decisions as tech leads and architects? How do we approach all these massive changes? What plan do we have to follow if we need to upgrade safely our applications without postponing their roadmap? Tough questions right?
There is not a silver bullet here. The only think I ‘ve learnt all these years is to stay proactive and move forward with careful steps trying to maintain a balance. We definitely need to embrace all these new features and make the most out of new React versions but we don’t have to go crazy.
React.StrictMode
Thankfully, React team has taken care of that too so with StrictMode we can maintain stability for huge codebases while upgrading small parts in steps.
StrictMode is a tool for highlighting potential problems in an application. It won’t render anything but it will throw a bunch of errors in console when it finds some issues for the children components.
Errors will look like this:
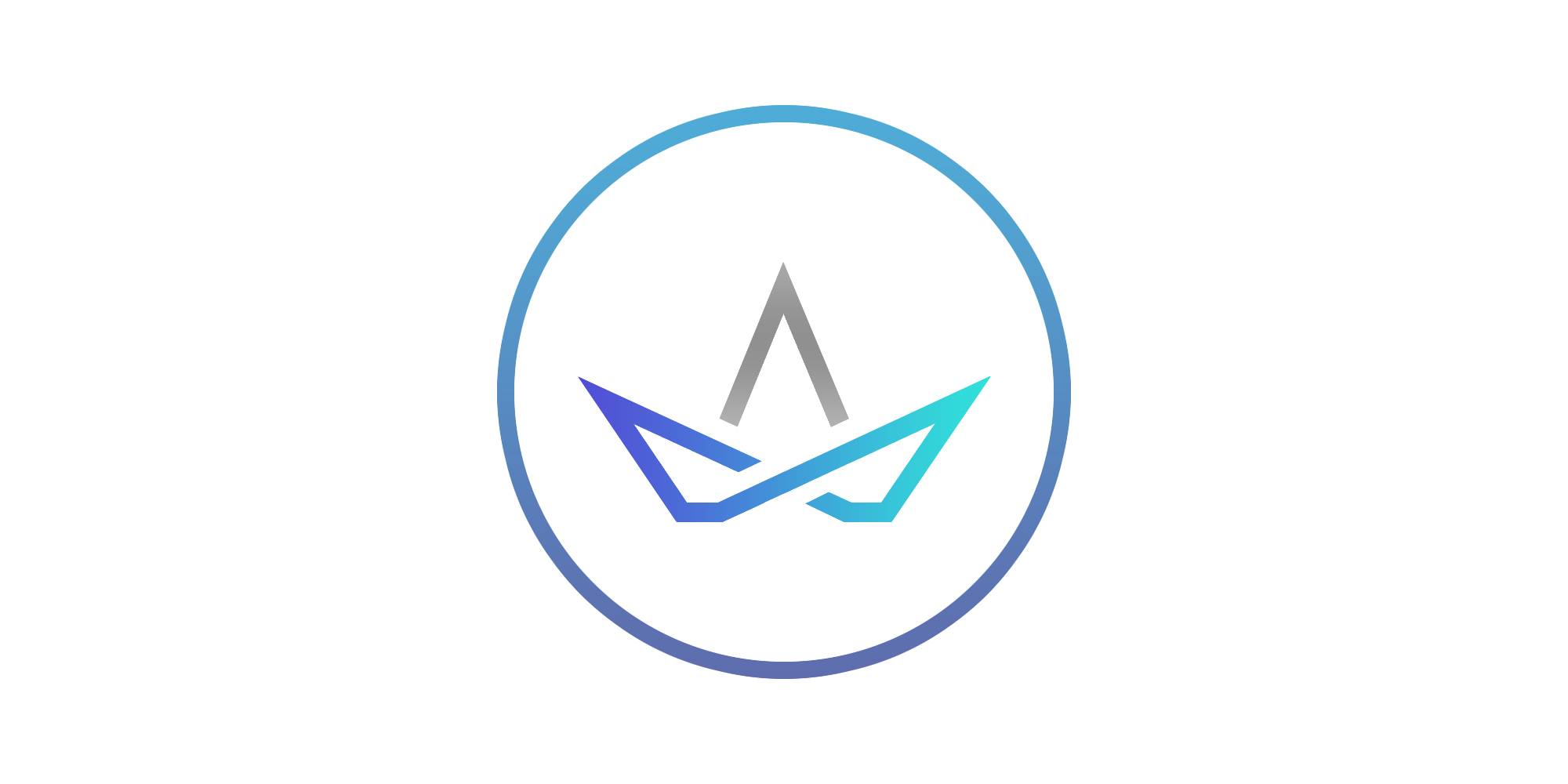
We can easily wrap the whole app with it. This approach might be ok for small codebases:
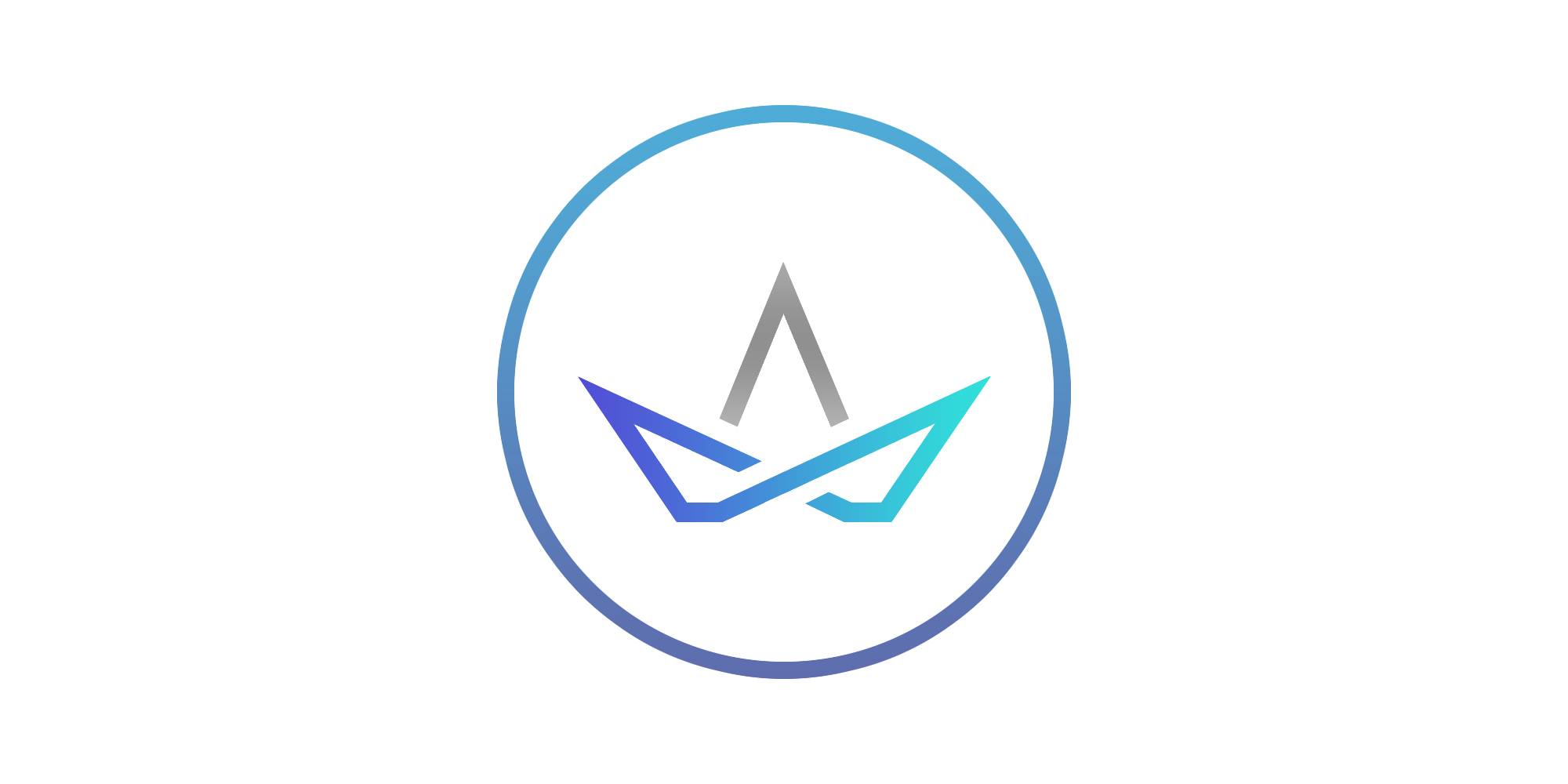
Of course, this is definitely not the best way to take advantage of it in large applications. Obviously, the console is going to get crazy. Especially if we are using it while trying to upgrade an older React version to v16.
In that case, I highly recommend to use it carefully in specific parts or even components of the codebase so you can upgrade them in steps:
Some issues we expect to spot with React.StrictMode:
- Identifying components with unsafe lifecycles
- Warning about legacy string ref API usage
- Warning about deprecated findDOMNode usage
- Detecting unexpected side effects
- Detecting legacy context API
Be aware that this will also throw errors for all the 3d party libraries used by the components you are trying to upgrade. Some times this is even more important so you check if all these 3d party libraries are following React progress. Maybe this will be an indication that some of them are not maintained at all. If this is the case, then I suppose it is about time to get rid of them or maybe start contributing to them or even fork them.
Code that runs twice in development mode
React.StrictMode, in order to be efficient and avoid potential problems by any side-effects, needs to trigger some methods and lifecycle hooks twice. These are:
- Class component constructor method
- The render method
- setState updater functions (the first argument)
- The static getDerivedStateFromProps lifecycle
- React.useState function
It is important to know that React.StrictMode doesn’t run in production mode so you don’t need to worry about all these double runs at all.
Conclusion
React 16 has tons of goodies that can help us into writing safer and more robust code and provide definitely even more business value in the end-product. Concurrent rendering is on its way and we definitely want to stay on track.
By definition, it is wrong to upgrade a client-side application in one big step especially if we are talking about a really big codebase that needs some great amount of time and involves a humongous team effort. React.StrictMode is provided so we can move to the modern era of React by upgrading our components in steps with confidence while adding new features and business value to our product. Cheers!!
Marios Fakiolas
Full-stack JavaScript lover, happy husband, proud father ?
Software Engineering Manager / Frontend Head at @omilialtd